1. 如何读取摄像头
在图像处理中,读取视频并进行处理是必不可少的操作,在OpenCV中,读取摄像头的视频所用到的主要函数为 capture()
。
①VideoCapture类的构造函数:
1 2 3
| VideoCapture::VideoCapture() VideoCapture::VideoCapture(const string& filename) VideoCapture::VideoCapture(int device)
|
②读取摄像头视频的函数:
1 2
| VideoCapture& capture.read(Mat& image)
|
此函数用于捕获视频的每一帧,并返回刚刚捕获的帧如果没有视频帧被捕获,返回false。
读取摄像头视频的示例程序如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| void MyDemo::video_Demo(Mat& image) { VideoCapture capture(0); Mat frame; while (true) { capture.read(frame); flip(frame, frame, 1); if (frame.empty()) { break; } imshow("Video", frame); char k = waitKey(10); if (k == 'q') { break; } } capture.release(); }
|
2. 视频的存储
视频的存储所用到的是 VideoWriter
类。所使用到的类属性和方法如下
1 2 3 4 5 6 7
| bool open( const string& filename, int fourcc, double fps, Size frameSize, bool isColor=true );
|
其中fourcc编码格式可选参数如下:
参数 |
编码格式 |
CV_FOURCC(‘P’,‘I’,‘M’,‘1’) |
MPEG-1 |
CV_FOURCC(‘M’,‘J’,‘P’,‘G’) |
motion-jpeg |
CV_FOURCC(‘M’, ‘P’, ‘4’, ‘2’) |
MPEG-4.2 |
CV_FOURCC(‘D’, ‘I’, ‘V’, ‘3’) |
MPEG-4.3 |
CV_FOURCC(‘D’, ‘I’, ‘V’, ‘X’) |
MPEG-4 |
CV_FOURCC(‘U’, ‘2’, ‘6’, ‘3’) |
H263 |
CV_FOURCC(‘I’, ‘2’, ‘6’, ‘3’) |
H263I |
CV_FOURCC(‘F’, ‘L’, ‘V’, ‘1’) |
FLV1 |
-1 |
弹出一个编码器选择框 |
保存摄像头视频的程序:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| void MyDemo::video_Demo(Mat& image) { VideoCapture capture(0); int frame_width = capture.get(CAP_PROP_FRAME_WIDTH); int frame_height = capture.get(CAP_PROP_FRAME_HEIGHT);
VideoWriter writer; int fourcc = writer.fourcc('D', 'I', 'V', 'X'); writer.open("E:/Program/OpenCV/vcworkspaces/opencv_452/img/test.mp4", fourcc, 30, Size(frame_width, frame_height), true);
Mat frame; while (capture.isOpened()) { capture.read(frame); flip(frame, frame, 1); if (frame.empty()) { break; } writer.write(frame); imshow("Video", frame); char k = waitKey(33); if (k == 'q') { break; } } capture.release(); writer.release(); }
|
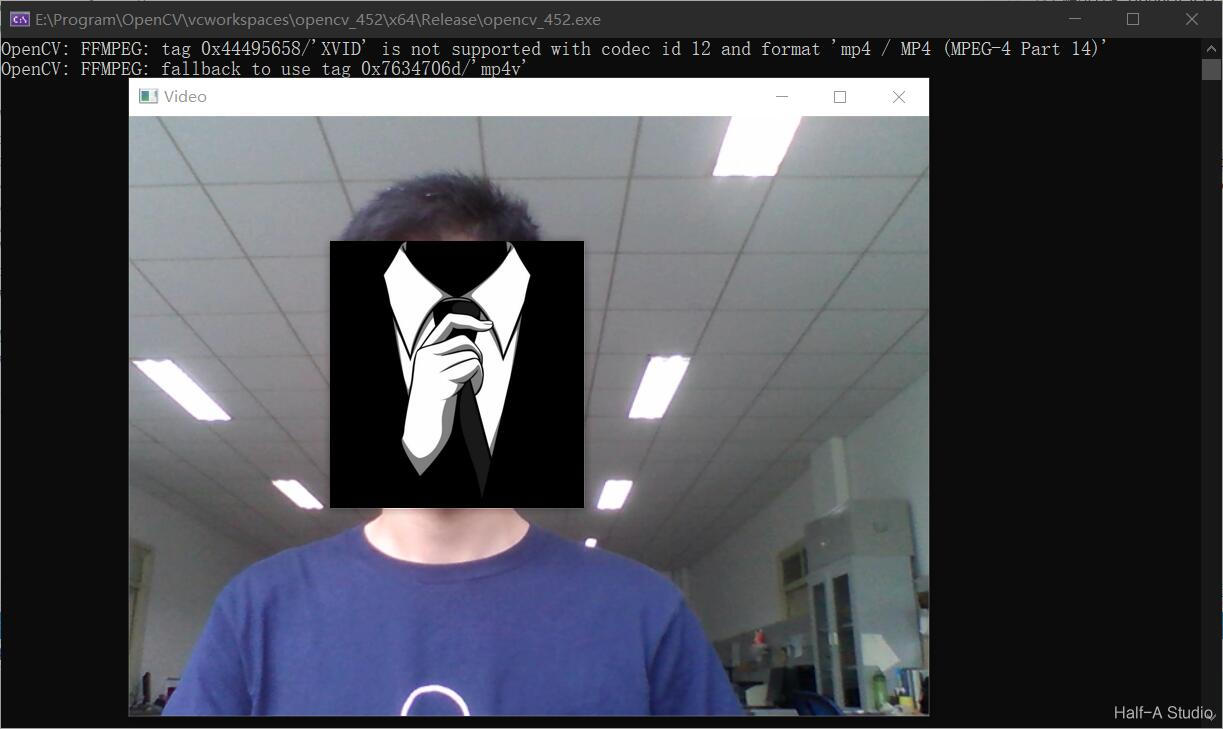